Liferay provides lots of configurations under Instance settings for OOTB features. We can add our own configuration in the Liferay control panel so that we can save configurable values for our project. Here you will learn about how we can implement custom configuration in Liferay 7.2.
Prerequisites
- Java
- Liferay portal 7/7.x
Environment Requirement
- JDK
- Eclipse
- MySQL
1) Create module project with below classes
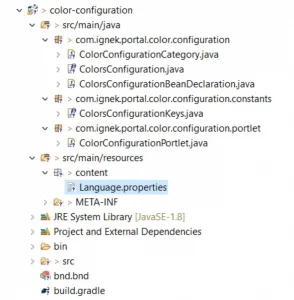
2) Create a Java interface
Its interface is used to configure the Liferay form application.
// ColorsConfiguration.java
@ExtendedObjectClassDefinition(category = "colors-configuration", scope = ExtendedObjectClassDefinition.Scope.COMPANY)
@Meta.OCD(id = ColorsConfigurationKeys.COLORS_CONFIGURATION, localization = "content/Language", name = "colors-configuration")
public interface ColorsConfiguration {
@Meta.AD(deflt = "descriptive",
name = "colors",
optionLabels = { "%White", "%Red", "%Yellow" },
optionValues = {"white", "red", "yellow" },
required = false)
public String colors();
}
Meta.OCD
Registers this class as a configuration with a specific id. The ID must be the fully qualified configuration class name.
Meta.AD
Specifies optional metadata about the field, such as whether it’s a required field or if it has a default value. Note that if you set a field as required and don’t specify a default value, the system administrator must specify a value in order for your application to work properly. Use the default property to specify a default value.
3) Create category and section
If you don’t want the default sections and categories, you can make your own by implementing the ConfigurationCategory interface.
// ColorConfigurationCategory.java
package com.ignek.portal.color.configuration;
import ...
@Component(service = ConfigurationCategory.class)
public class ColorConfigurationCategory implements ConfigurationCategory {
private static final String CATEGORY_ICON = "cog";
private static final String CATEGORY_KEY = "colors-configuration";
private static final String CATEGORY_SECTION = "color configuration";
@Override
public String getCategoryIcon() {
return CATEGORY_ICON;
}
@Override
public String getCategoryKey() {
return CATEGORY_KEY;
}
@Override
public String getCategorySection() {
return CATEGORY_SECTION;
}
}
Note : please edit the Language.properties located in module project’s location /src/main/resources/content/Language.properties
category.colors-configuration=Colors Configuration
colors-configuration=Colors Configuration
category-section.color-configuration=Color Configuration
4) Create a configuration bean declaration
It’s used to register your configuration class.
// ColorsConfigurationBeanDeclaration.java
package com.ignek.portal.color.configuration;
import ...
@Component(
immediate = true,
service = ConfigurationBeanDeclaration.class)
public class ColorsConfigurationBeanDeclaration implements ConfigurationBeanDeclaration {
@Override
public Class> getConfigurationBeanClass() {
return ColorsConfiguration.class;
}
}
After registering with a ConfigurationBeanDeclaration, you’re ready to use your Configuration in module projects.
Deploy the configuration module and now you can see your configuration in your Control Panel → Configuration → Instance Settings.


Now you can fetch custom configuration values in your custom portlet using the following code.
// ColorConfigurationPortlet.java
package com.ignek.portal.color.configuration.portlet
@Component(
...
)
public class ColorConfigurationPortlet extends MVCPortlet {
private Log log = LogFactoryUtil.getLog(this.getClass().getName());
private ConfigurationProvider configurationProvider;
@Reference(unbind = "-")
protected void setConfigurationProvider(ConfigurationProvider configurationProvider) {
this.configurationProvider = configurationProvider;
}
@Override
public void render(RenderRequest renderRequest, RenderResponse renderResponse)
throws IOException, PortletException {
ThemeDisplay themeDisplay = (ThemeDisplay) renderRequest.getAttribute(WebKeys.THEME_DISPLAY);
ColorsConfiguration colorsConfiguration;
try {
colorsConfiguration = configurationProvider.getCompanyConfiguration(ColorsConfiguration.class,themeDisplay.getCompanyId());
System.out.println("colorsConfiguration----------->" + colorsConfiguration.colors());
} catch (ConfigurationException e) {
log.error(e);
}
super.render(renderRequest, renderResponse);
}
}
This will print the color configuration value you have saved from Liferay Control Panel.