Introduction
When confronted with the task of uploading a substantial volume of data into a Liferay object, it can become a time-consuming process due to the necessity of manually inputting all the data.
In a professional context, consider the scenario where thousands of individuals fill out a form, and the objective is to store this data within a Liferay object.
In this case, the efficient approach is to read the data from a CSV file, extract the relevant information, and subsequently populate it into the Liferay object.
Prerequisites:
- CSV File
- Java JDK 11
- Entry Object
Target
- Retrieve the Object and add the dependencies to your module.
- Read the CSV File and convert its entries into a JSON object.
- Retrieve the Entries from the JSON object.
- Add the JSON object’s data entries to the Liferay Object.
Implementation
1) Retrieve the Object and add the dependencies to your module:
- Here we are using an MVC portlet to do this.
- We have an object named ‘Data Upload.’ In this step, we are retrieving the Object Definition.
//Fetching Object for storing data in it's field.
ObjectDefinition dataUploadObj = null;
try {
dataUploadObj = ObjectDefinitionLocalServiceUtil.getObjectDefinitionByExternalReferenceCode("liferay-data-upload", PortalUtil.getDefaultCompanyId());
} catch (PortalException e) {
e.printStackTrace();
}
- Add these dependencies to your build.gradle file.
// build.gradle
compileOnly group: "org.osgi", name: "org.osgi.service.component.annotations"
compile group: "com.opencsv", name: "opencsv", version: "3.8"
implementation group: 'org.apache.poi', name: 'poi', version: '3.15'
implementation group: 'org.apache.poi', name: 'poi-ooxml', version: '3.15'
implementation group: 'org.apache.commons', name: 'commons-csv', version: '1.5'
implementation group: 'javax.mail', name: 'mail', version:
2) Read the CSV File and convert its entries into a JSON object:
- Before reading the CSV, we need to create a File Object and specify the file path within it.
// Defining File Path
String filePath = "C:\\Users\\jvardey\\Documents\\CLM-CSV-.csv";
File csvFile = new File(filePath);
- After obtaining the file, we proceed to validate whether the fetched path is a valid CSV file path and ensure that it is not null.
// Validating the CSV file is null or not
if (Validator.isNotNull(csvFile)) {
if (csvFile.getName().contains(".csv")) {
} else {
_log.info("Uploaded File is not CSV file.Your file name is ----> ");
}
}
- We are now creating the FileInputStream object and passing it to the InputStreamReader object.
- The InputStreamReader object will be instrumental in creating a CSV Parser. With the assistance of the CSVParser, we read the records from the CSV file.
- During each iteration, we utilize the createJsonObject method to generate a JSON object for each row in the CSV file.
// Creating JSONArray Object
JSONArray csvDataArray = JSONFactoryUtil.createJSONArray();
try (InputStream targetStream = new FileInputStream(csvFile);
InputStreamReader isr = new InputStreamReader(targetStream);) {
CSVFormat csvFormat = CSVFormat.newFormat(',').withIgnoreEmptyLines().withTrim(true);
CSVParser csvParser = csvFormat.parse(isr);
if (csvParser != null) {
JSONObject rowObject = null;
for (CSVRecord record : csvParser) {
rowObject = JSONFactoryUtil.createJSONObject();
for (int j = 0; j < record.size(); j++) {
rowObject.put(String.valueOf(j), record.get(j));
}
csvDataArray.put(rowObject);
}
}
} catch (IOException e) {
e.printStackTrace();
throw e;
}
3) Retrieve the Entries from the JSON object:
if (Validator.isNotNull(csvDataArray)) {
System.out.println("Data Array Length ===> " + csvDataArray.length());
for (int i = 1; i < csvDataArray.length(); i++) {
try {
Map map = new HashMap();
JSONObject jsonObject = csvDataArray.getJSONObject(i);
ServiceContext serviceContext = new ServiceContext();
serviceContext.setCompanyId(PortalUtil.getDefaultCompanyId());
if (Validator.isNotNull(jsonObject.getString("0"))) {
map.put("address", jsonObject.getString("0"));
}
if (Validator.isNotNull(jsonObject.getString("1"))) {
map.put("city", jsonObject.getString("1"));
}
if (Validator.isNotNull(jsonObject.getString("2"))) {
map.put("country", jsonObject.getString("2"));
}
if (Validator.isNotNull(jsonObject.getString("3"))) {
map.put("name", jsonObject.getString("3"));
}
ObjectEntryLocalServiceUtil.addObjectEntry(dataObj.getUserId(), 0,
dataObj.getObjectDefinitionId(), map, serviceContext);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Suppose we have only four desired fields. To retrieve the entries from the JSON object, we simply iterate through the JSON object in a loop and store all the entries in a HashMap.
4) Add the JSON object’s data entries to the Liferay Object:
ObjectEntryLocalServiceUtil.addObjectEntry(dataObj.getUserId(), 0,dataObj.getObjectDefinitionId(), map, serviceContext);
Now, we are adding the JSON object entries to our Liferay object.
We previously fetched the Liferay object in the first step, where we intended to add the entries.
After completing this process, you will find the result in the Object as follows:
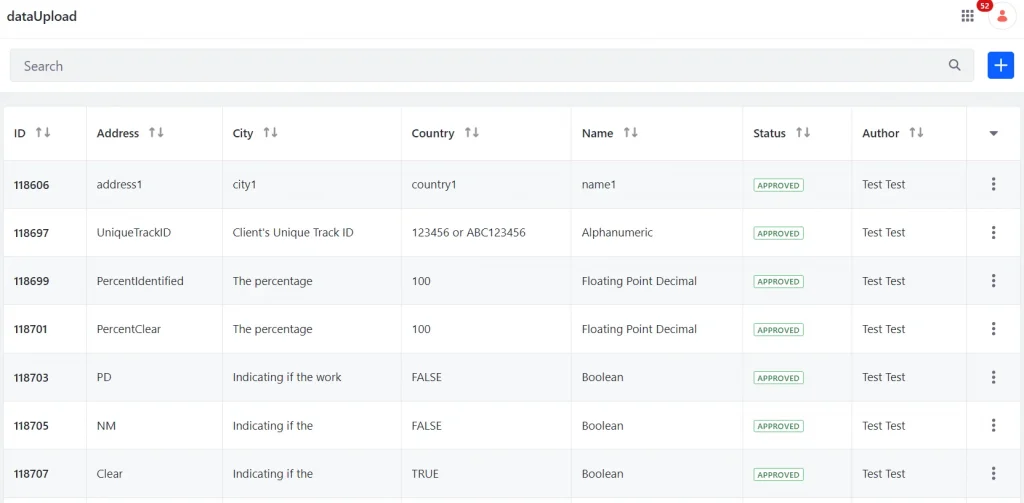